Build an AI assistant on your GitHub repo

Build an AI assistant on your GitHub repo with data persisted locally. Make sure you’ve installed the ddn CLI and have an Anthropic API key.
Make sure you’re on the latest version of the ddn CLI.
ddn update-cli --version v2.12.0-alpha.2
Clone the repo
Clone using http:
git clone https://github.com/hasura/example-promptql-github.git
cd example-promptql-github
or ssh:
git clone [email protected]:hasura/example-promptql-github.git
cd example-promptql-github
Set up your .env file
cp .env.example .env
Add Anthropic API key to .env
Get an api key from https://console.anthropic.com/settings/keys
...
ANTHROPIC_API_KEY=<your-anthropic-api-key>
To use an OpenAI key instead, you’ll have to set OPENAI_API_KEY
in your .env file and change the environment variable
LLM
to openai
in the compose.yaml file.
Add GitHub API token to .env
Head over to https://github.com/settings/tokens and create an API token.
...
GITHUB_API_TOKEN=<GITHUB_API_TOKEN>
This token only needs read access to the repo you are interested in.

Choose the org/repo
Head to app/connector/github/index.ts
and change the org name and the repo name to something you’d like:
// index.ts
...
const manager = new GitHubIssueSyncManager('<org-name>', '<repo-name>');
if (!process.env.GITHUB_API_TOKEN) {
...
Setup DDN project
ddn project init
Start PromptQL
First build your supergraph
ddn supergraph build local
Then bring up the PromptQL API server, the engine and the connector
ddn run docker-start
You’ll notice in amongst your Docker logs that your github synchronization has started.
Docker logs
[2024-01-01 12:00:02] Fetching repository metadata…
[2024-01-01 12:00:03] Syncing issues and pull requests…
[2024-01-01 12:00:04] Loading repository contents…
[2024-01-01 12:00:05] GitHub sync complete
If the specified repo has many issues or comments it may take some time to get them all and you may be rate limited. That’s ok, you can go ahead and try PromptQL without the process having fully finished yet.
If you notice some logs regarding GitHub permissions, check that your GitHub API token has the correct permissions for the repo you’re trying to access.
Open the PromptQL playground
In another terminal, run
ddn console --local
Log in with your Hasura account on the browser page that loads up.
Ask questions about your GitHub repo
The console is a web app hosted at console.hasura.io that connects to your local PromptQL API and data sources. Your data is processed in the DDN PromptQL runtime but isn’t persisted externally.
Head over to the console and ask a few questions about your GitHub repo.
> What are the open pull requests?
> What kind of questions can I ask?
Start from scratch?
If you made a mis-step or want to start from scratch, simply restarting the docker containers will reset the app. However, this will also lose any data which may have been loaded.
ddn run docker-start
Deploy and share your PromptQL app
Create a supergraph build
Heads up, this takes a good 5-6 minutes to run while the connector is provisioned on DDN.
ddn supergraph build create
Open the PromptQL playground
The PromptQL playground opens in your browser.
ddn console
Enter your Anthropic API key. The key will be saved in your DDN project.
Act on your data!
Ask questions about your GitHub repo.
> Who are the most prolific contributors?
> How much time does it take to close an average issue?
Remember, you can always ask additional follow up questions, or provide instructions iteratively to explore and act on your data.
Read more at the PromptQL Guide.
Share your PromptQL app
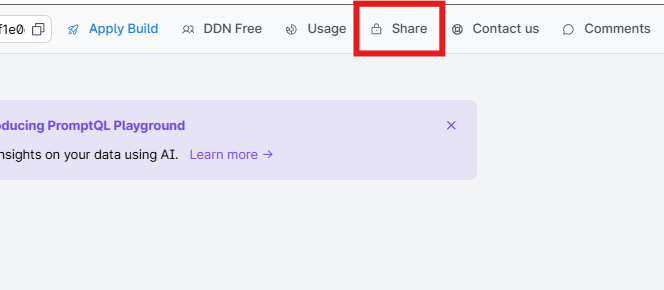
Users with any access level, including “Read only” can access your promptQL app. Read only users cannot modify your project or invite additional users.
You can also choose “Request Access” so that anyone who arrives at the project URL can request access.
Edit and extend
If you’d like to tweak how this app works check out the app/connector/github/functions.ts
file and especially the
GitHubIssueSyncManager
function.